FIEA Technical Artist Portfolio
As the title suggests, this blog shall contain some of my favorite pieces of code/artwork that I’d be submitting for my Technical Artist Portfolio (for FIEA) in no particular order.
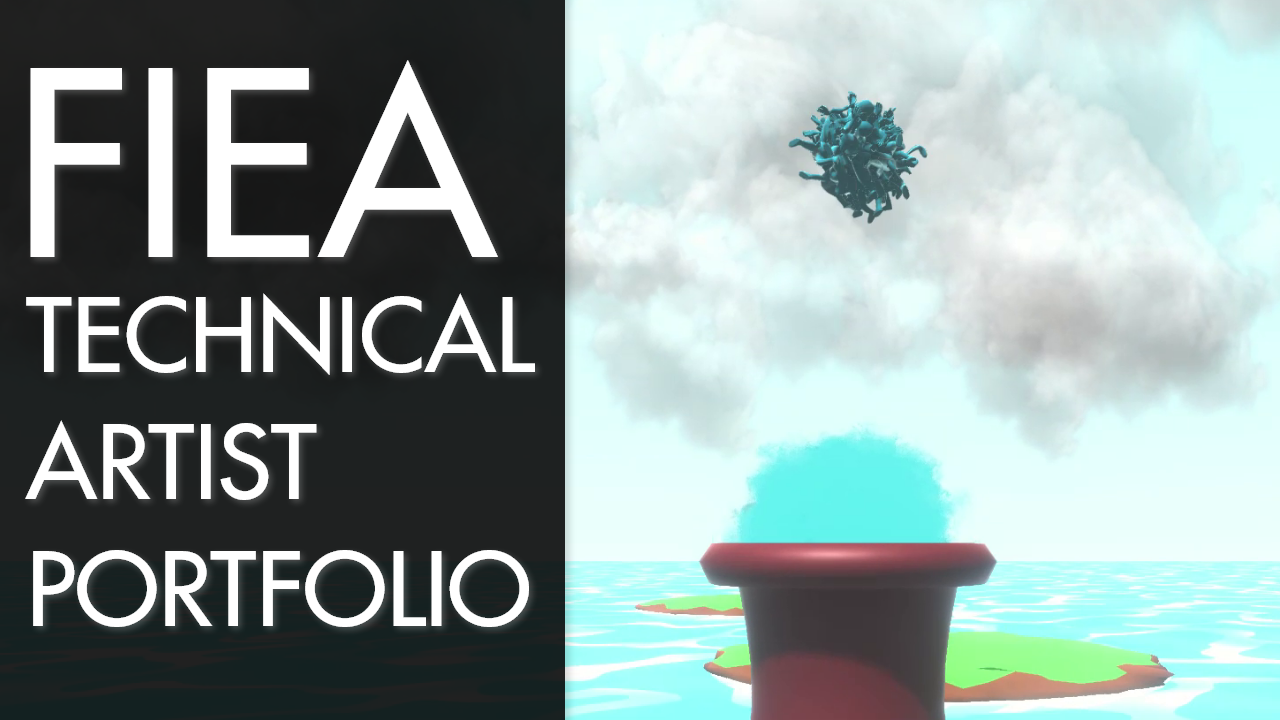
1.0 2D Lighting System - Unity
- While competing for the Brackeys Game Jam 2021.1, we decided to make a top-down atmospheric 2D game as they were trending a lot on itch.io back then.
- Back when we started working on this project, Unity didn’t have any rendering pipeline that supported 2D lighting, so I developed my own lighting system for it. This was primarily done to add to the atmospheric look of the game.
- The detailed documentation and source code can be found here.
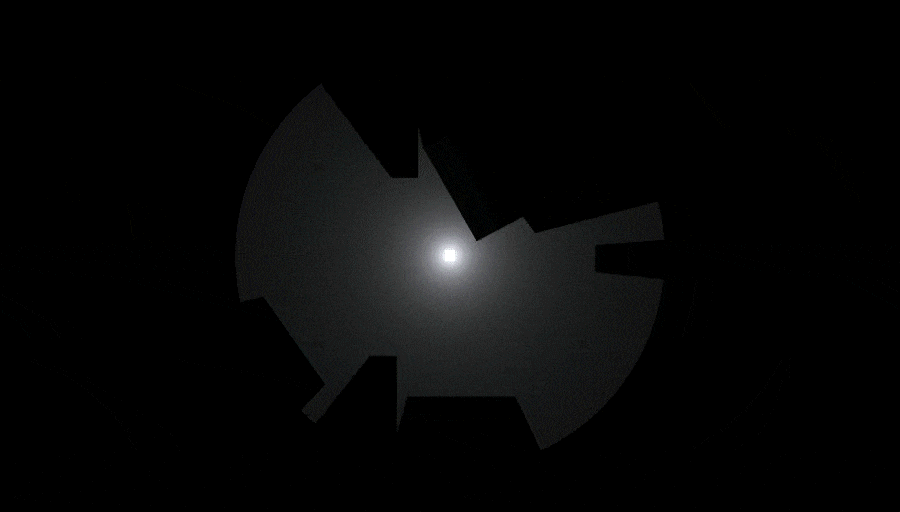
The first iteration
- The first iteration uses the Unity’s low level Graphics Library (gl) to draw rays emerging from the player.
- An Unlit Shader that supports both transparency and vertex colors is used as the ray’s material to make the light rays feel natural.
- The environment is scripted to react to the lighting.
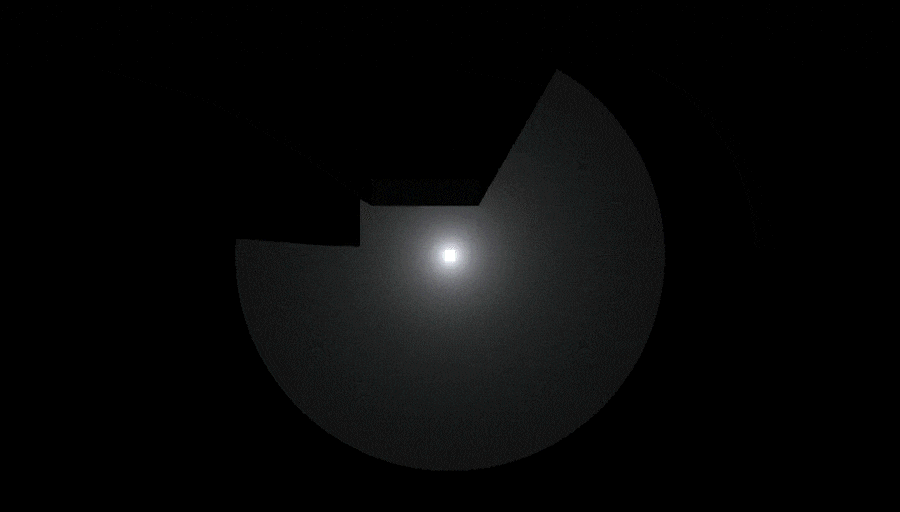
The second iteration (optimized)
- The previous lighting method was very inefficient with time complexity of
O(n)
as the loop had to run 3600 times every frame with a step size of 0.1. - I solved this issue by detecting the edges of nearby objects, casting rays at them, and then filling the space by generating mesh between them in the second iteration.
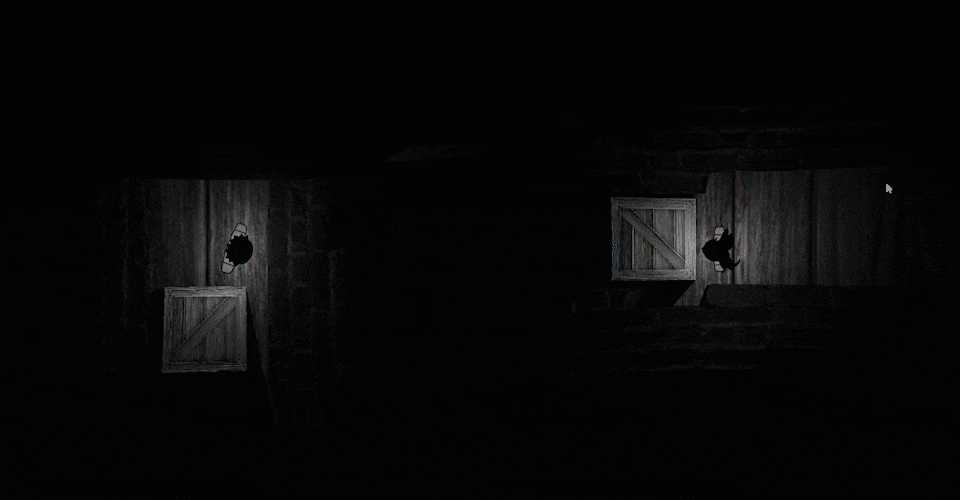
In-game look
- This is how the lighting finally looked in the game, with some post-processing thrown on top of it. You can try it yourself here.
2.0 ASCII Line Art - p5js
- After getting an experience as a tech artist at FIEA this summer, I was confident this was the track that I’d be applying to.
- To provide a testimony to it, I decided to make ASCII Line art for all professors and mentors this Teacher’s Day.
- I designed 52 of these in total and made one for Prof. Chris Roda, Prof. Rick Hall, and Prof. Ron Weaver as well.
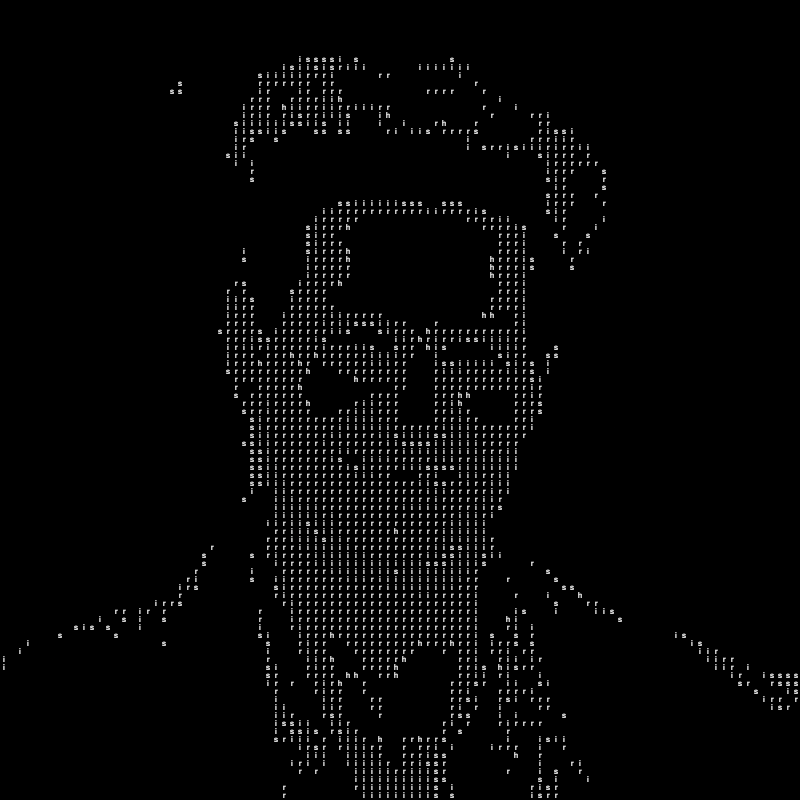
Chris Sir
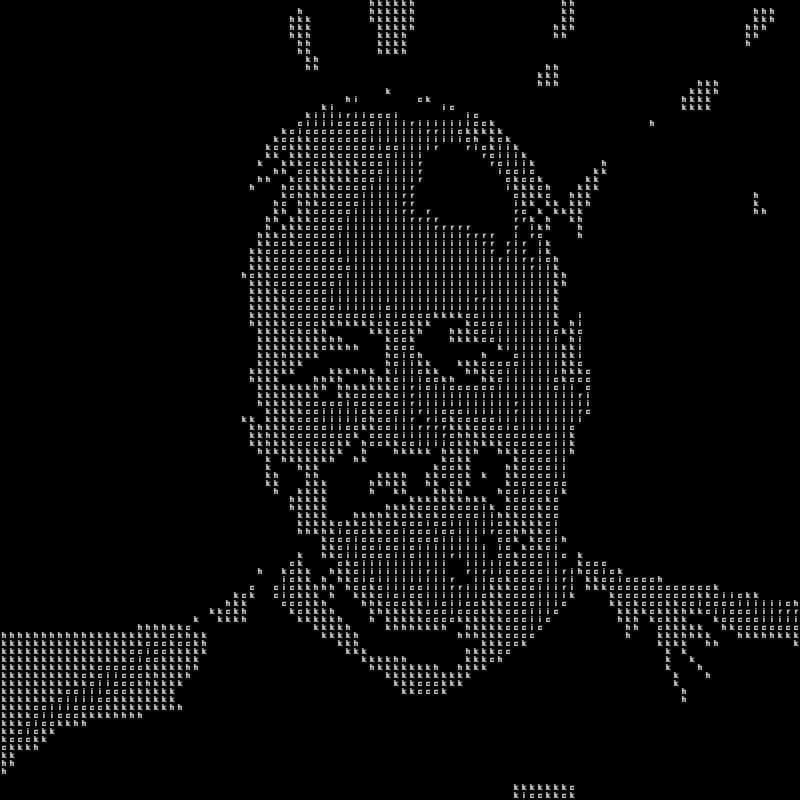
Rick Sir
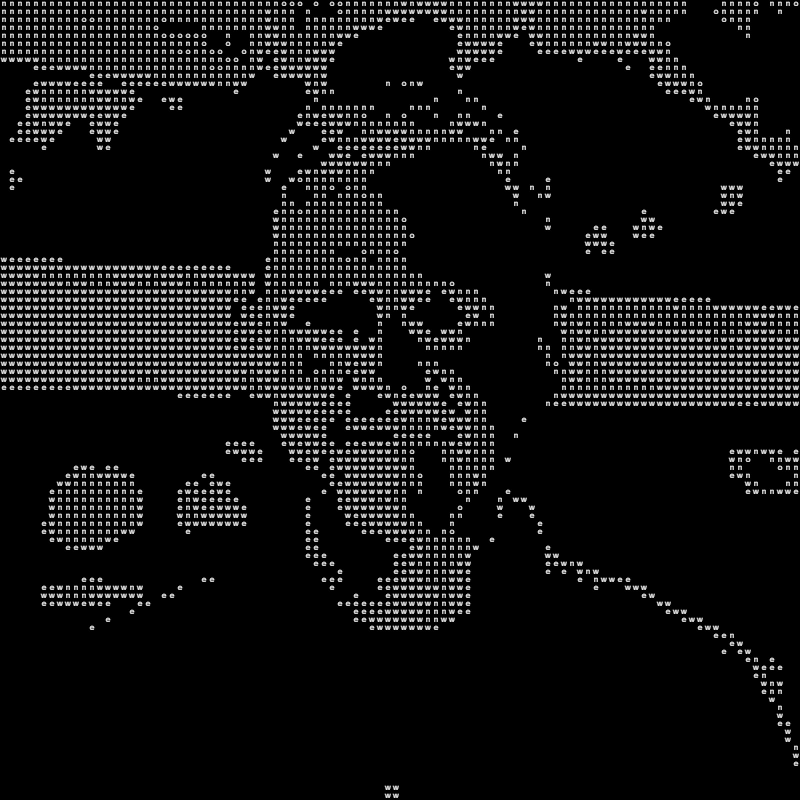
Ron Sir
- These were made using p5js by detecting changes in the overall RGB values of different parts of images.
//text to be used in the image
const name = 'chrisroda';
function draw() {
//setting background to black
background(0);
let w = width / img.width;
let h = height / img.height;
img.loadPixels();
//iterating through pixels and assigning letters based on average rgb values
for (let i = 0; i < img.width; i++) {
for (let j = 0; j < img.height; j++) {
const pixelIndex = (i + j * img.width) * 4;
const r = img.pixels[pixelIndex + 0];
const g = img.pixels[pixelIndex + 1];
const b = img.pixels[pixelIndex + 2];
const avg = (r + g + b) / 3;
noStroke();
fill(255);
const len = name.length;
const charIndex = floor(map(avg,0,255,len,0));
textSize(w);
textAlign(CENTER, CENTER);
//edge detection (hardcoded)
if(avg > 100 && avg < 180)
text(name.charAt(charIndex), i * w + w * 0.5, j * h + h * 0.5);
}
}
}
- If you zoom onto the images (by clicking on them), you’ll find out that the pixels of the images are converted into letters of the name of the person that it’s made for.
- Many of these images were converted into DXF format and engraved on acrylic sheets to be gifted to the professors.
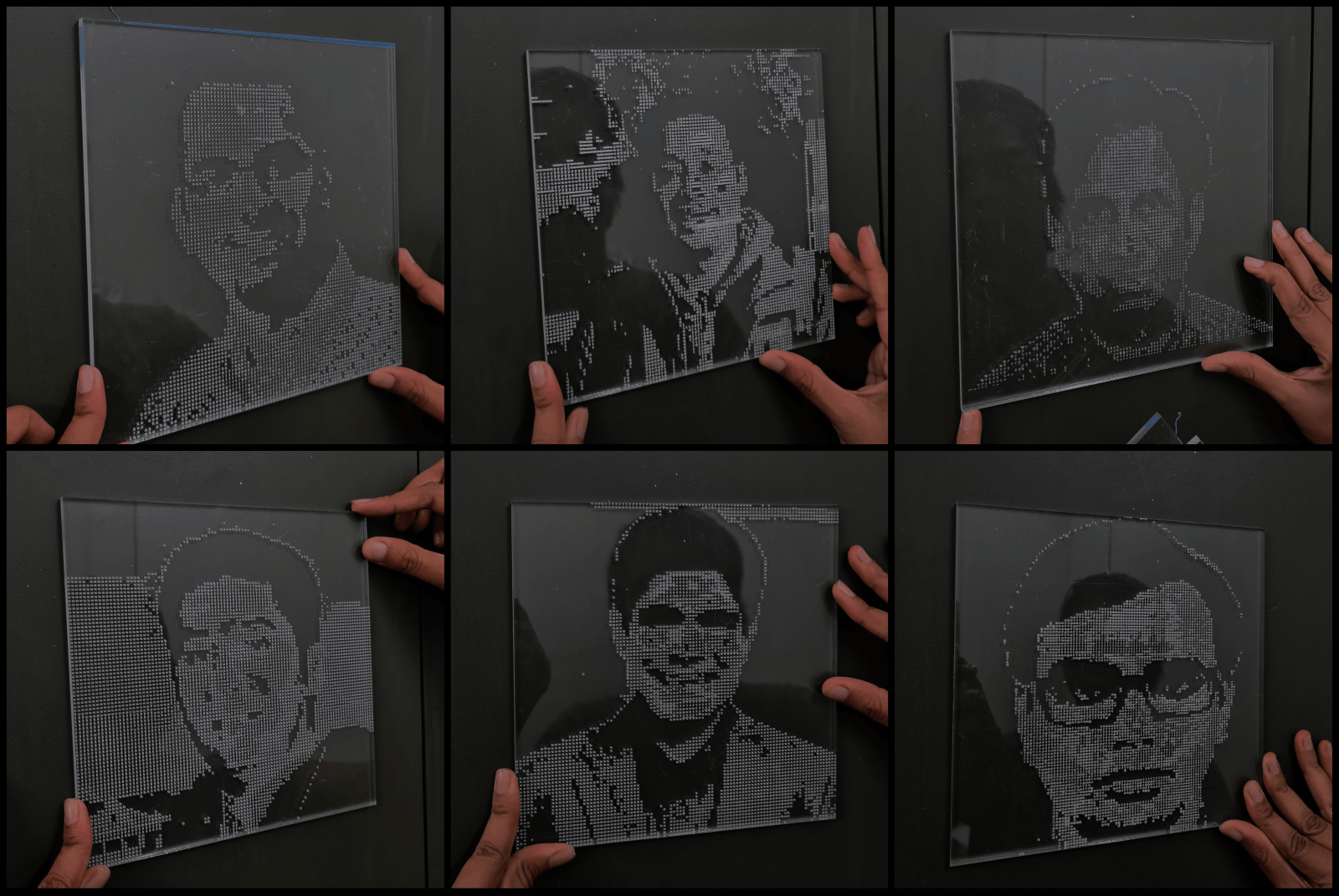
Engraved Images
3.0 Raymarched Clouds
- While working on Human Canon prototype for CrazyLabs, I encountered the classic problem of optimizing volumetric clouds for low-end devices (especially mobile).
- While doing my research over the internet, I came across this video about raymarched clouds by Sebastian Lague.
- This video eventually drew my attention towards raymarching, and eventually, I wrote my own raymarcher for Unity. You can find the project here. The HLSL (Shader) files can be found here.
- Further, I followed this article about making raymarched volumetric clouds in Unreal engine to develop my own version of the same in Unity.
Raymarched Clouds in Unity
4.0 Raymarched 4D Environment
- I further extended the raymarching engine to render 3D sections of Four-dimensional objects like HyperCube, HyperSphere and DuoCylinder.
Raymarched 4D Objects in Unity
- The 4D shape distance functions—
//Hypercube
float Hypercube(float4 p, float4 b)
{
float4 d = abs(p) - b;
return min(max(d.x,max(d.y,max(d.z,d.w))),0.0) + length(max(d,0.0));
}
//Hypershpere
float Hypersphere(float4 p, float s)
{
return length(p) - s;
}
//Duo Cylinder
float DuoCylinder( float4 p, float2 r1r2) {
float2 d = abs(float2(length(p.xz),length(p.yw))) - r1r2;
return min(max(d.x,d.y),0.) + length(max(d,0.));
}
5.0 Snowstorm System - Soul Shard
- I assisted the 19 Souls on Board team at FIEA as a technical artist for their capstone project Soul Shard. The game is getting processed by Steam and will be out soon!
- As a part of this engagement, one of my tasks was to develop a snowstorm system for the yard area that’s situated in the middle of the map by looking at a reference video.
- The detailed documentation and source code can be found here.
- To simulate a natural looking snowstorm, I decided to divide the snowstorm into three subsystems—
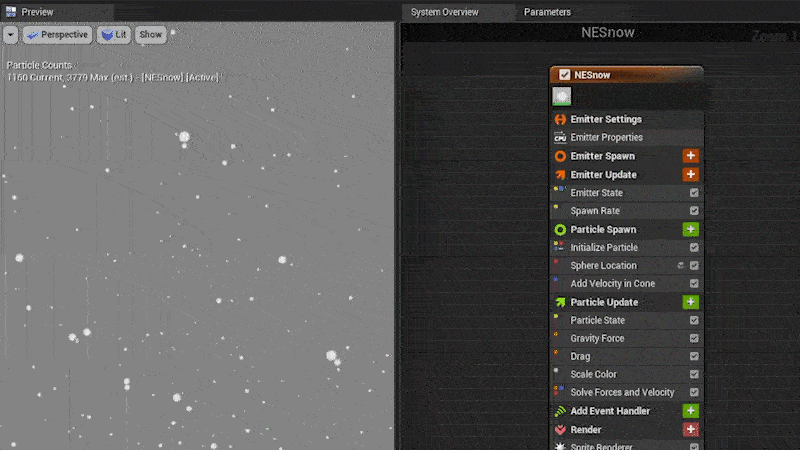
Snow Subsystem
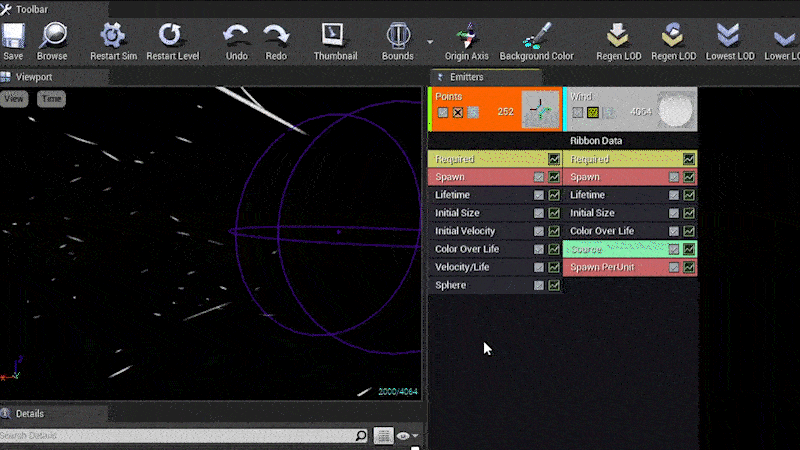
A Wind Subsystem
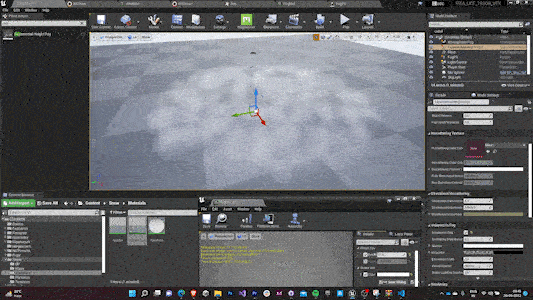
A Fog Subsystem
- Putting these together after proper scaling gave the following result—
Snowstorm System Final In-Game Look
6.0 Digital Art - Hypercasual Concept Pitches
- As a part of the PPP (Pay-Per-Prototype) deal by CrazyLabs, I was expected to deliver Concept Pitches of various Hypercasual ideas and they approved the best ones for me to develop.
- To make the digital art mockups for these, I used Procreate on iPad.
- These are the mockups for the game Tilt Paint (the original concept pitch can be found here)—
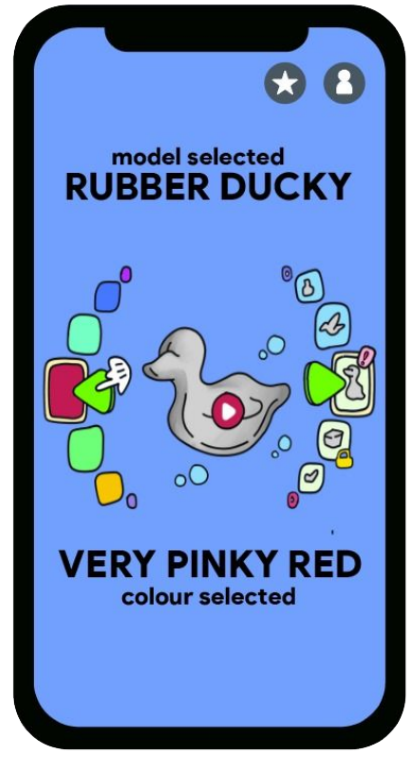
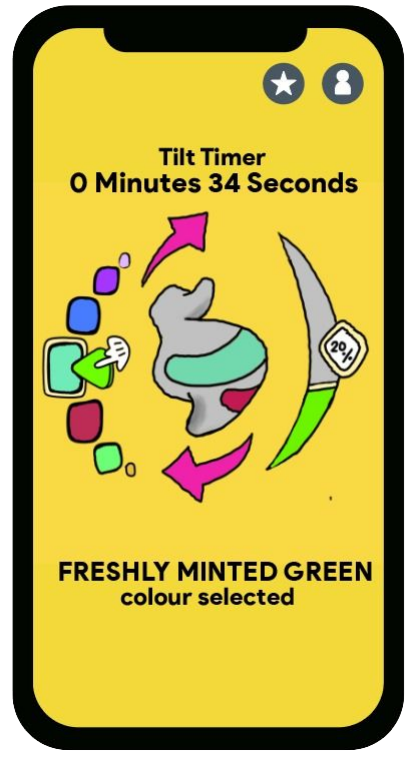
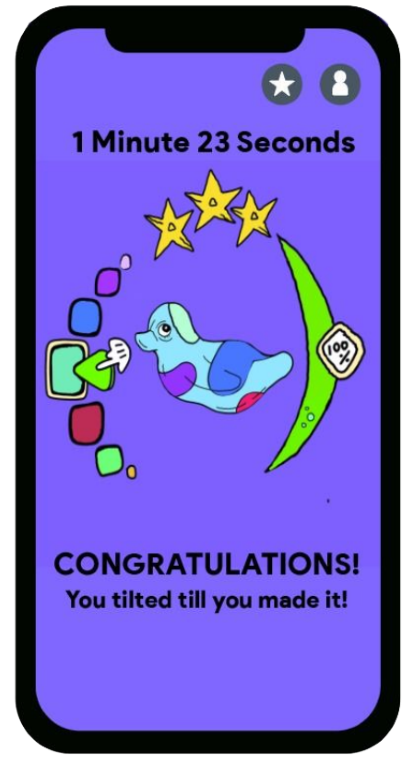
- Some mockups were rendered in Blender too. Take for instance the mockup of Body Crack ASMR (the original concept pitch can be found here)—
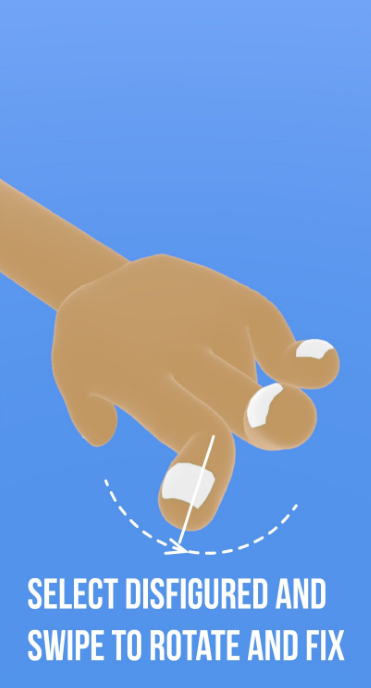
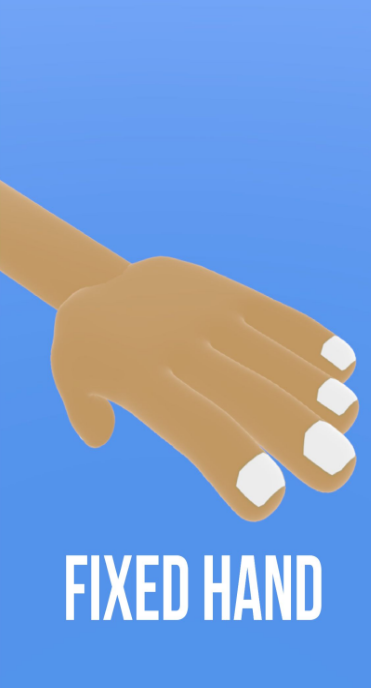
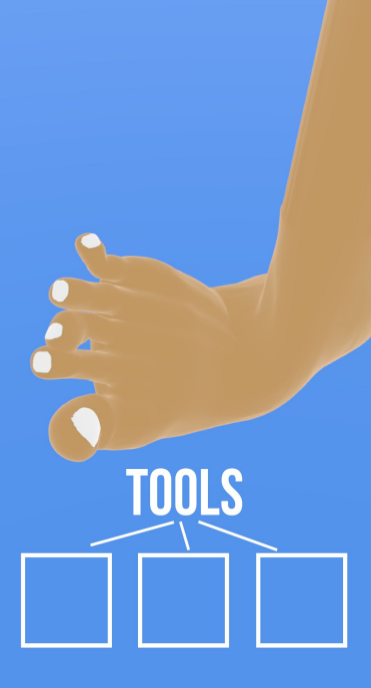
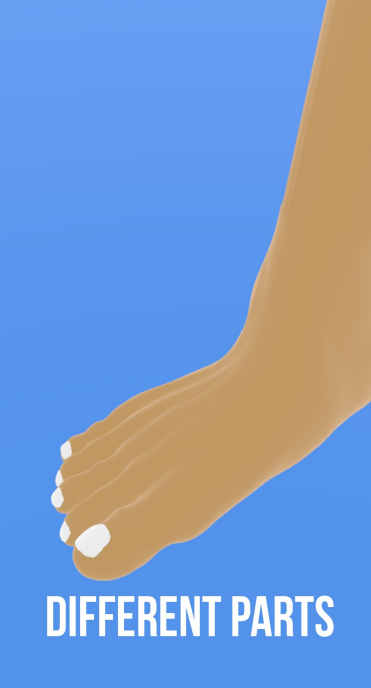
- The comprehensive list and source files for all the 30 concept pitches delivered can be found here by scrolling to the
concept pitches
section.
7.0 Retro Shader & Post Processing - Are Ya Winning, Son?
- While participating in the GMTK Game Jam 2020, we worked on a retro-styled game where the AI won’t let you win.
- To add to the retro theme, we decided to use shaders and post-processing effects so that the game feels as if it’s being played on a CRT TV.
- This was achieved by using Post-Processing & a distorted render texture layered on top of it.
- You can play the game here and watch its devlog here.
8.0 Pixel Art - Doge to the Moon
- While competing for the Opera GX Game Jam, I worked on Pixel Art of various characters of our game Doge to The Moon.
- All of these Pixel Arts were made using the GameMaker Studio 2’s Sprite Editor.
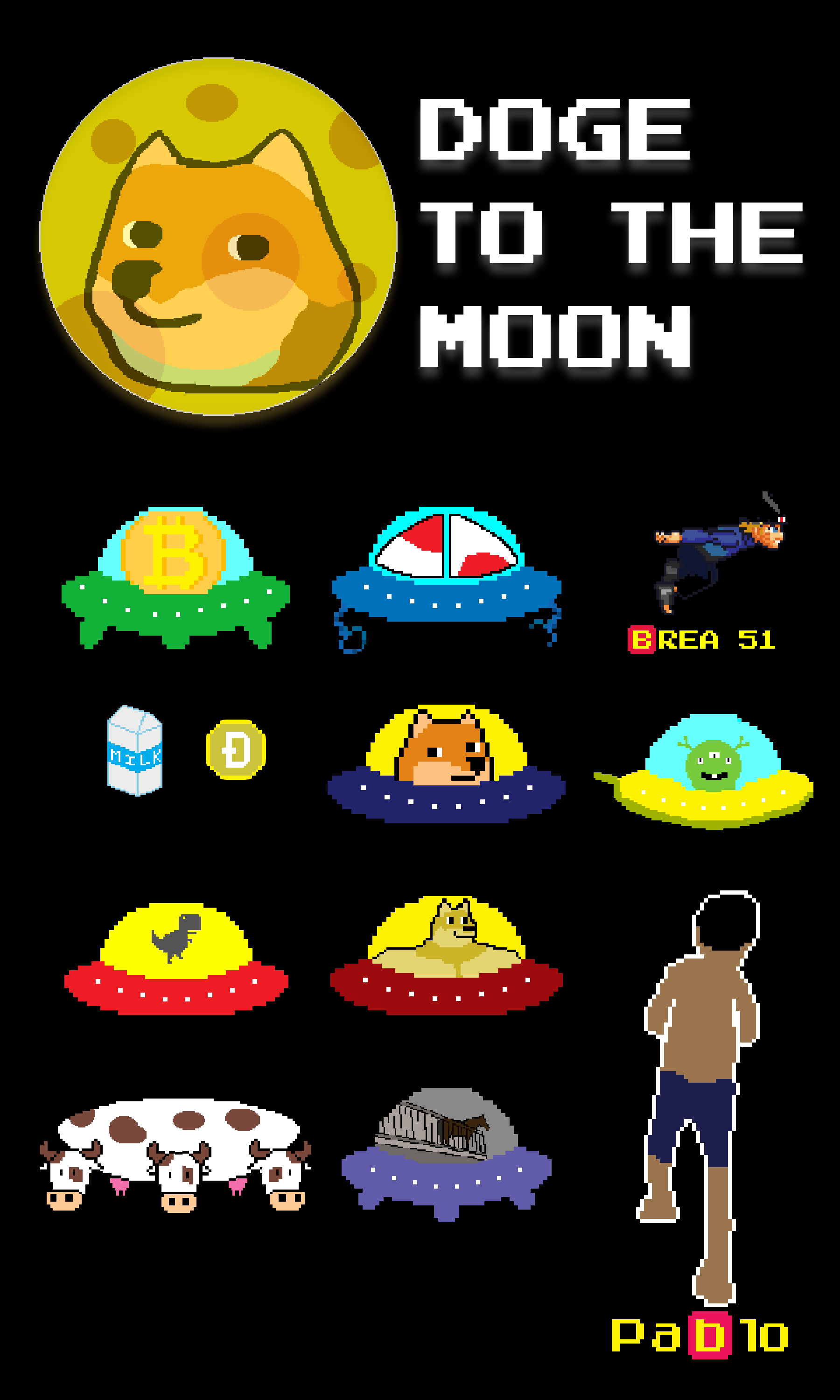
All Pixel Arts
- All of these assets also had their glowing counterpart to mimic the animation of getting hit.
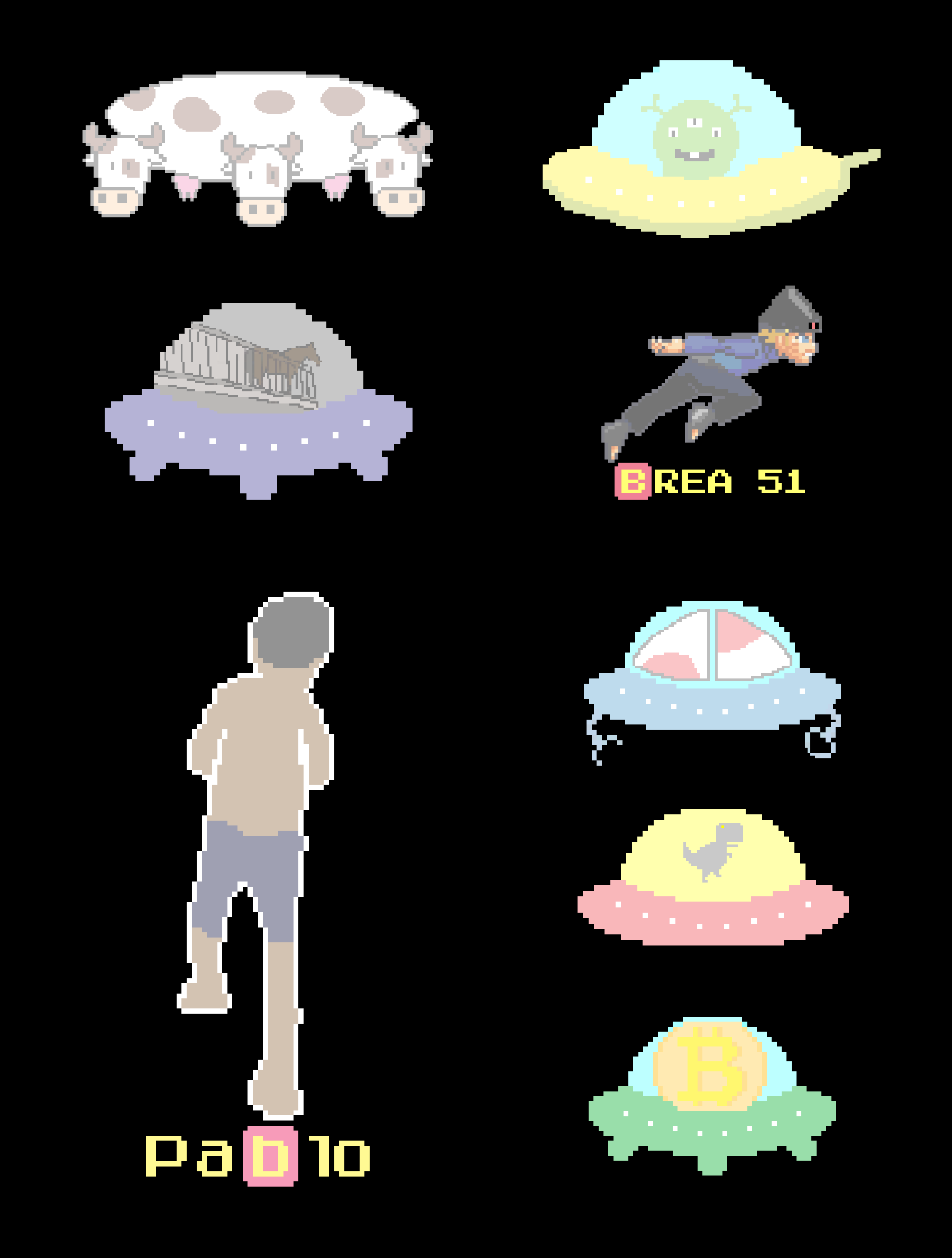
Glowing Pixel Arts
- Most of the sprites were converted into sprite sheets to animate the sprites with slight variations in each slice of the sprite sheet.
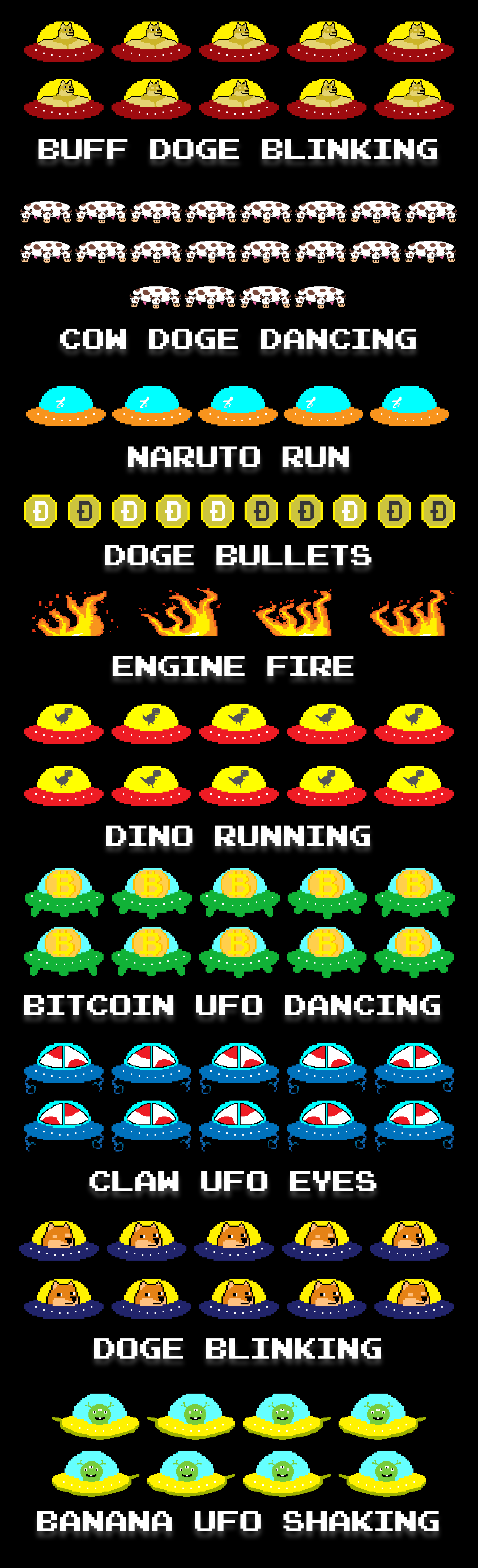
Animation Sprite Sheets
- Apart from these sprites, I also designed the background images (starts and the space) and made them scroll vertically at different speeds to give a parallax effect.
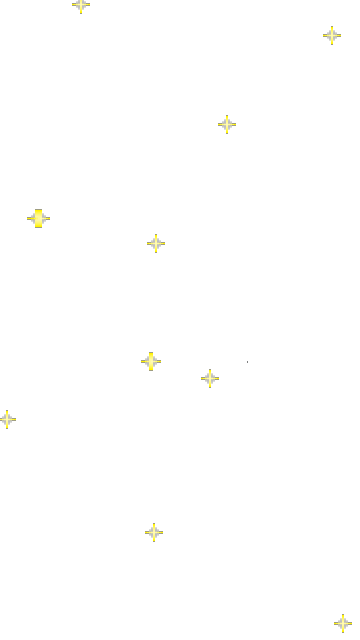
Stars Section
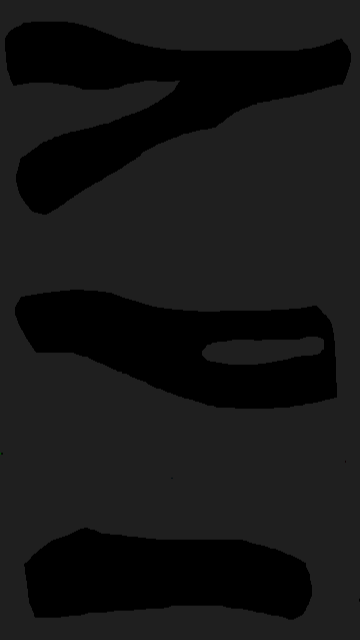
Background Section
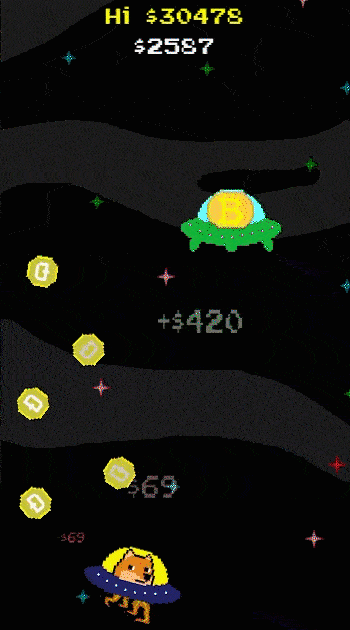
Final In-Game Look
9.0 VFX Graph in Unity
- I had to make a theme reveal video for a Game Jam that my college was hosting.
- I decided to utilize this opportunity and learn VFX graphs in Unity.
- VFX graph help simulate over a million particles in real-time as they use the parallel processing capabilities of GPU, unlike the default particle system that runs on CPU.
- Everything that you see in this video is made out of ‘2020’, which was the theme for the JAM itself.
10.0 Non-Euclidean Worlds in Unity
- I worked on various Non-Euclidean world simulations inside of Unity, especially after CodeParade announced that it’s technically impossible to develop Non-Euclidean worlds in Unity without low-level access to its Rendering Engine.
- The first simulation consisted of cameras rendering over a render texture in the opening of a tunnel and colliders that teleported the player between different worlds seamlessly, offering a non-euclidean illusion.
- The subsequent two simulations used multiple intersecting single-sided planes instead of a 3d mesh to give a non-euclidean look.
11.0 Terrain Sculpting - Map of India for AFPS
- I led a team of 25 individuals to develop an Indian-themed battle royale game.
- The top view of the map was sculpted to represent the Indian map.
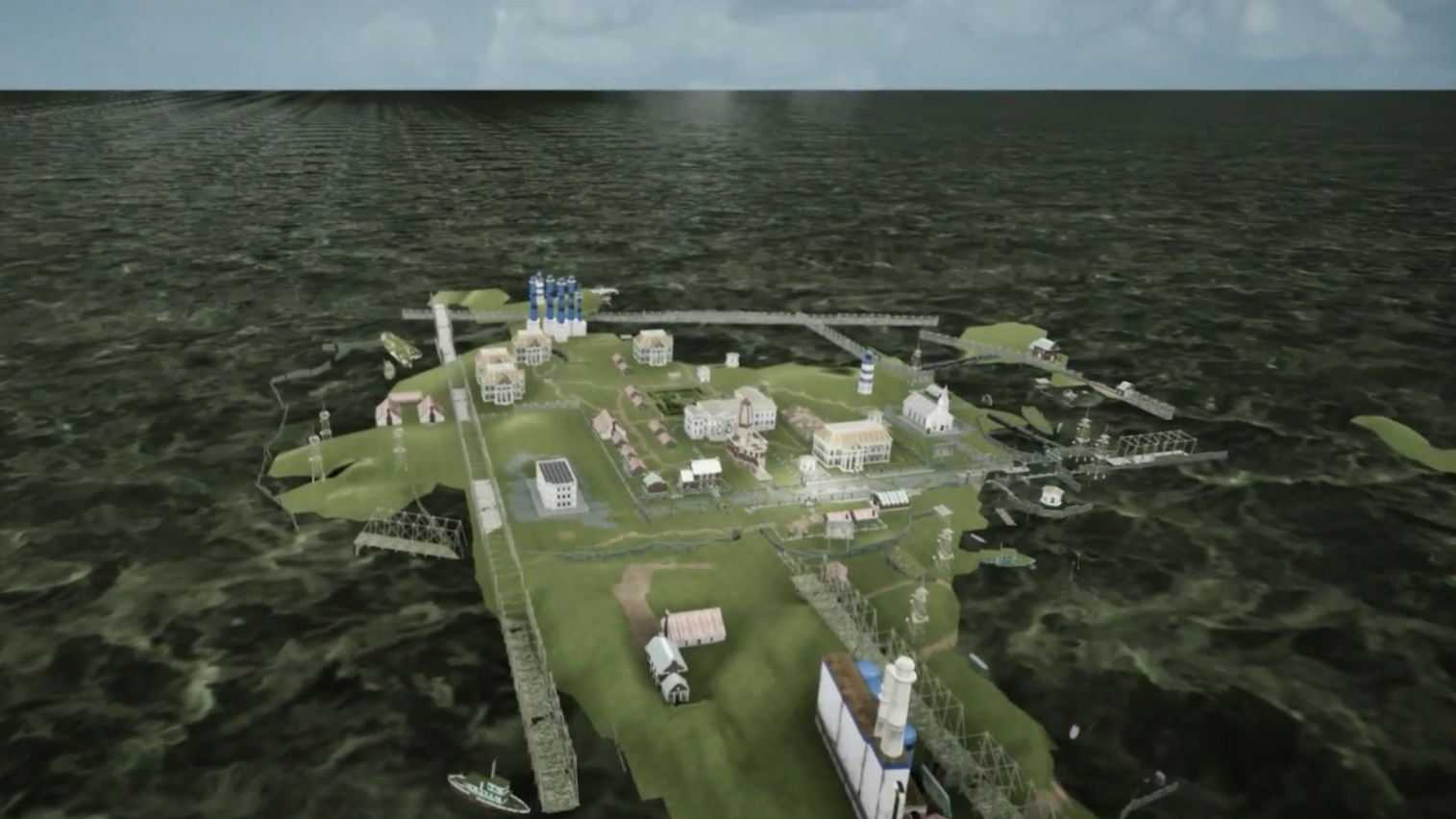
In-Game Look of the Map
- This terrain was sculpted using the Unity’s default Terrain Editor, and this is the timelapse video of developing this map-
12.0 Glowing Crack Material - Soul Shard
- I developed a material with glowing cracks governed by various parameters for Soul Shard.
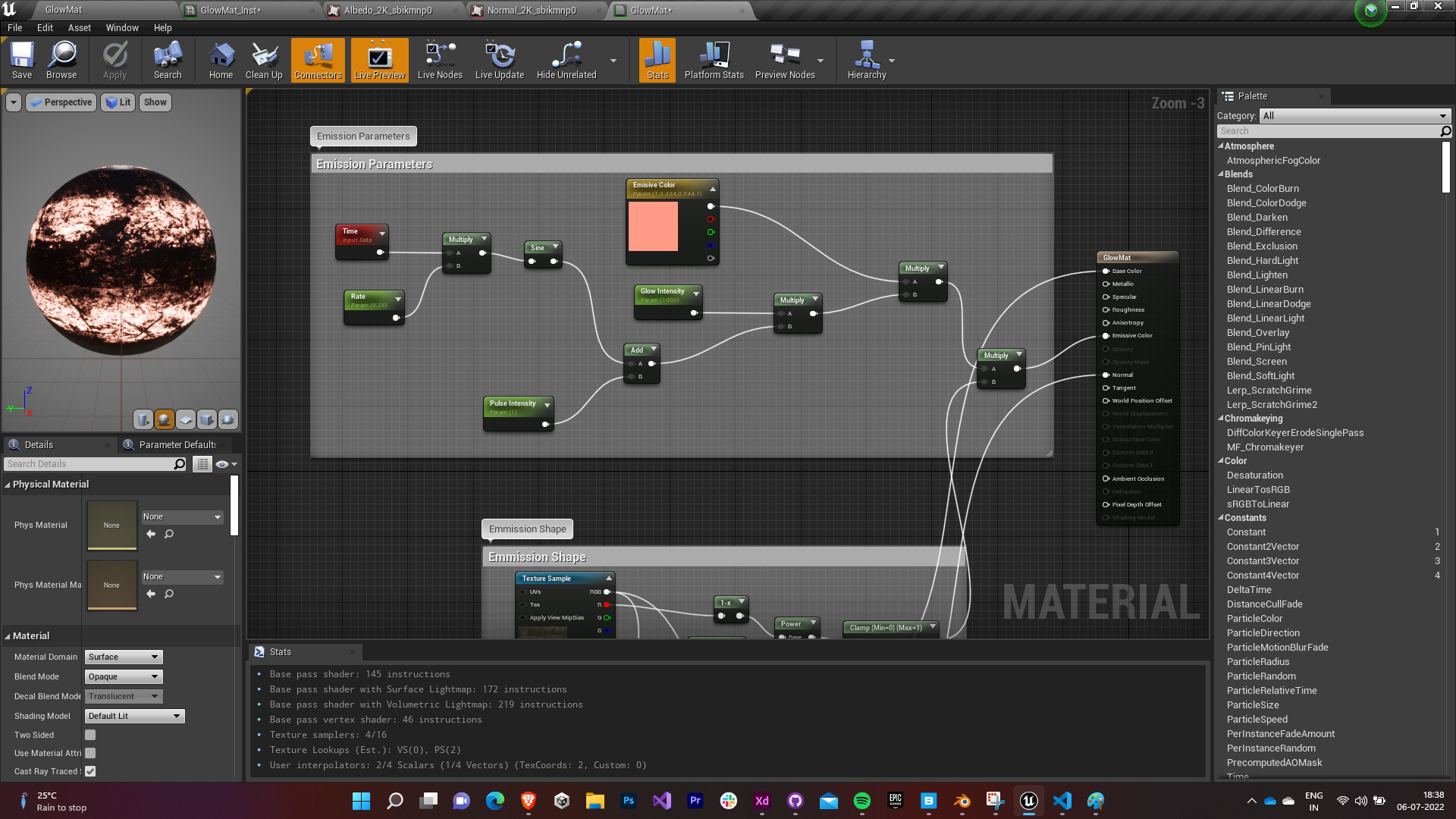
Emission Parameters
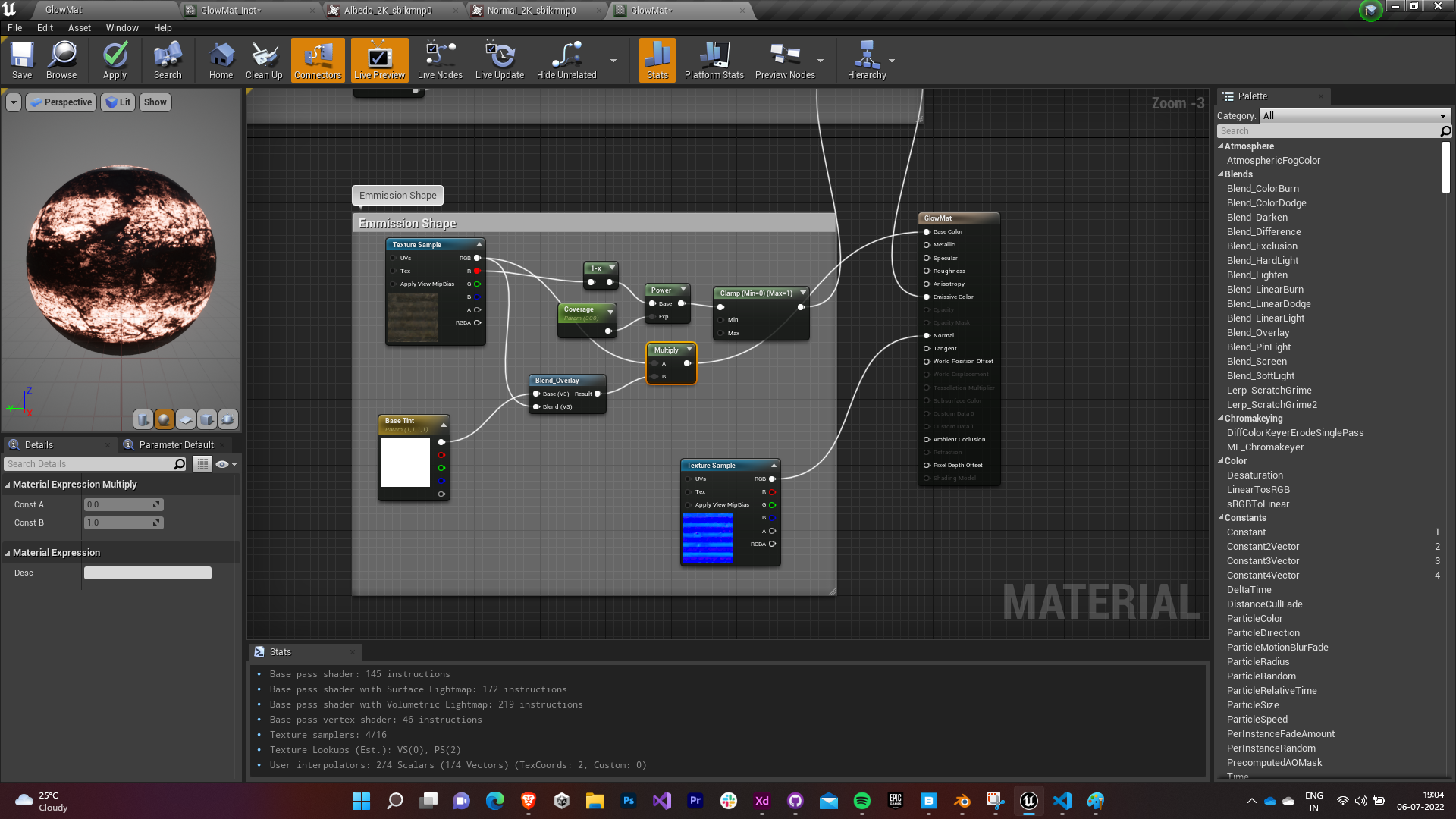
Emission Shape
- The material’s properties were governed by six parameters in total—
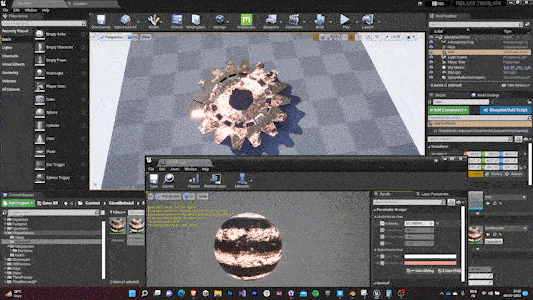
Coverage
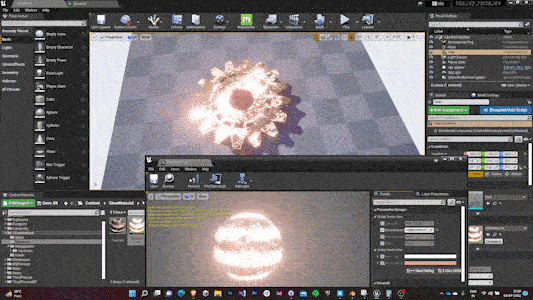
Glow Intensity
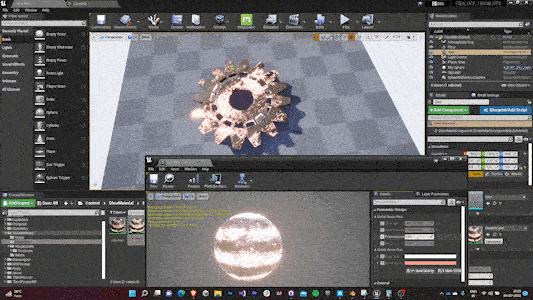
Pulse Intensity
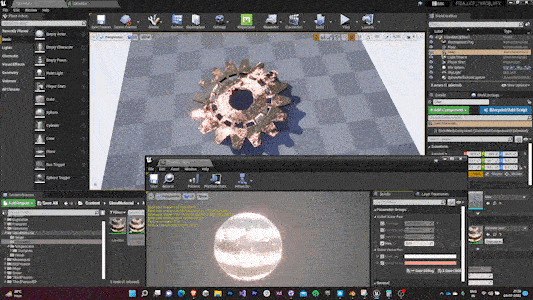
Rate
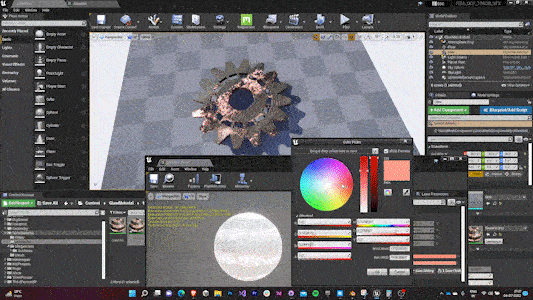
Emissive Color
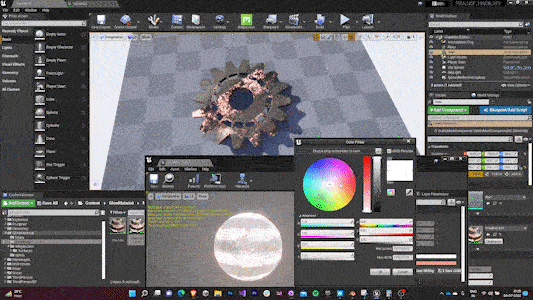
Base Tint